What is Bun.js
Introducing Bun.js: A Game-Changing JavaScript Toolkit/Runtime
Node.js has long been the preferred runtime for building scalable and high-performance web applications. But as the ecosystem evolves, so do the tools available to developers. Enter bun.js – a versatile, performance-focused toolkit that takes Node.js development to the next level. In this article, we'll explore the key features of bun.js that make it a must-have for Node.js experts.
What's JavaScript Runtimes
JavaScript runtime is a software environment that executes JavaScript code, and the specific runtime you use depends on the platform or type of application you are developing, whether it's for the web, server, mobile, or desktop. Each runtime provides its own set of APIs and capabilities tailored to its use case. It provides the necessary components and resources to run JavaScript code, such as the JavaScript engine, memory allocation, and event loop. The runtime is responsible for interpreting and executing JavaScript code, handling asynchronous operations, managing memory, and providing access to various APIs and libraries.
JavaScript Runtimes Types
- Web Browsers: The most common JavaScript runtime is the one built into web browsers like Chrome, Firefox, Safari, and Edge. These runtimes execute JavaScript code in web pages and interact with the DOM to create dynamic web applications.
- Node.js: Node.js is a server-side JavaScript runtime that allows you to run JavaScript on the server. It provides APIs for file system operations, network communication, and more, making it suitable for building web servers, command-line tools, and other server-side applications.
- Bun.js: Bun is a comprehensive JavaScript runtime and toolbox meticulously crafted for top-notch performance, offering an integrated bundler, a robust test runner, and a package manager fully compatible with Node.js.
- Deno: Deno is another server-side JavaScript runtime that is designed to be secure and efficient. It provides a runtime environment for JavaScript and TypeScript and comes with built-in utilities for file access, network communication, and sandboxing.
- React Native: React Native is a JavaScript runtime that allows you to build mobile applications for iOS and Android using JavaScript and React. It provides a bridge between JavaScript code and the native components of each platform.
- Electron: Electron is a framework for building cross-platform desktop applications using web technologies like HTML, CSS, and JavaScript. It embeds a Chromium-based web browser as its runtime for rendering the user interface.
Bun is a Fast JavaScript Runtime
Performance is a paramount concern in any web development project, and bun.js doesn't disappoint. This toolkit is designed to optimize your Node.js applications for maximum speed. It does this through various means, including aggressive tree shaking, minification, and a built-in cache system.
Bun is known for its speed due to its usage of the JavaScriptCore engine, whereas Node.js relies on the JavaScript V8 engine. The JavaScriptCore engine in Bun has been specifically optimized for quicker startup times, contributing to its enhanced performance.
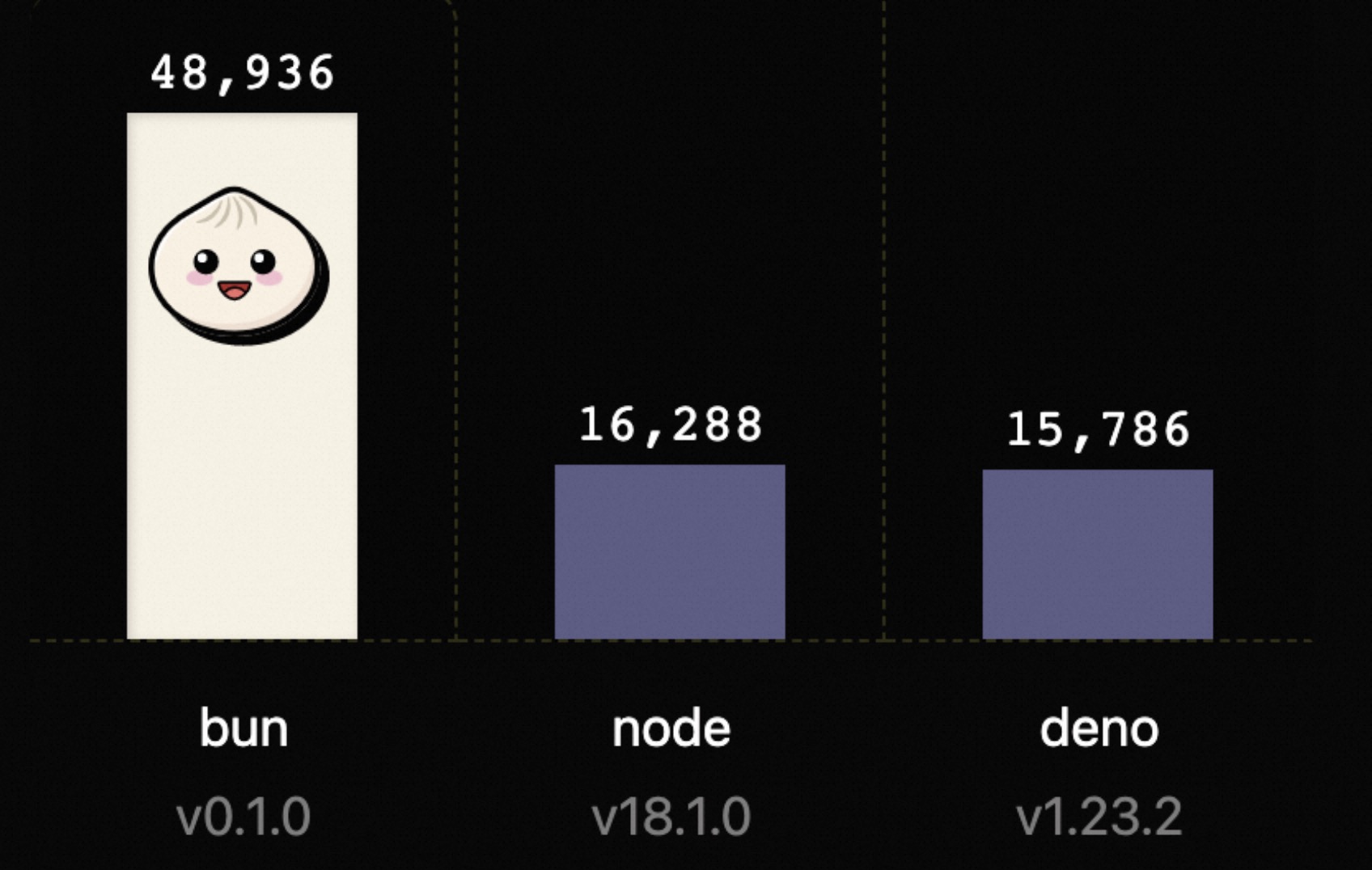
Comparison of server-side rendered React HTTP request per second (Linux AMD64)
TypeScript & JSX Support
For Node.js experts who prefer TypeScript or JSX in their projects, bun.js provides excellent support. You can seamlessly integrate TypeScript and JSX into your development workflow without the hassle of custom configurations. Bun.js understands your code, its dependencies, and its types, making it easier to catch errors during development rather than in production.
This level of support not only boosts developer productivity but also leads to more robust and maintainable codebases. With TypeScript and JSX, you can enjoy the benefits of static typing and improved tooling without any additional setup.
ESM & CommonJS Compatibility
The Node.js ecosystem has transitioned towards ECMAScript Modules (ESM), but not all existing codebases have made the switch. Bun.js bridges the gap between ESM and CommonJS, allowing you to use both module systems in your projects. This flexibility ensures that you can easily incorporate legacy code or third-party libraries that may still use CommonJS.
You can use
import
and require()
in the same file:import lodash from "lodash-es";
const _ = require("lodash");
By providing seamless compatibility, bun.js ensures that you're not locked into a specific module system and can gradually transition to ESM at your own pace.
Web-Standard APIs
Bun.js takes inspiration from web standards, which makes it easier for web developers to leverage their existing knowledge when working on Node.js projects. With bun.js, you can use familiar APIs, like window, document, and fetch, in your server-side code. This consistency simplifies the process of building isomorphic applications that share code between the server and client. By aligning with web standards, bun.js encourages code reuse and streamlines development for both front-end and back-end tasks.
Node.js Compatibility
As the Node.js ecosystem evolves, it's crucial to keep your projects up to date with the latest features and best practices. Bun.js is continuously updated to ensure compatibility with the latest Node.js releases. This means you can take advantage of new language features, performance improvements, and security enhancements without worrying about breaking changes.
Hot Reloading Without Killing Old Process
Hot reloading is a feature that enables developers to see immediate updates in their application as they make changes to the code. Unlike traditional development workflows that require manual restarts or refreshing the webpage, hot reloading automates this process, saving valuable time and effort.
The Magic of Bun's Hot Reloading: Bun, a powerful development tool, offers hot reloading as a convenient feature to streamline the development process. By running Bun with the
--hot
flag, you can enable hot reloading effortlessly. For example, running the command bun --hot server.ts
will trigger hot reloading for the specified "server.ts" file.Unlike other similar runtime such as Node.js, which typically perform hard restarts of the entire application process, Bun takes a different approach. It reloads the code without terminating the existing process. This means that ongoing HTTP and WebSocket connections remain intact, and the application's state is preserved. As a result, developers can seamlessly continue their work without interruptions or loss of data.
Highly-Customizable and Extensible Plugin
Bun's Plugin API is inspired by the popular esbuild tool, ensuring compatibility with existing esbuild plugins. This means that most esbuild plugins can be used directly within Bun, saving you time and effort in adapting them to your workflow. Whether you want to introduce custom transformations, optimizations, or any other functionality provided by esbuild plugins, Bun's plugin system allows for a seamless integration.
Get Started With Bun
Install Bun:
npm install -g bun
Implements a simple HTTP server via Bun:
const server = Bun.serve({
port: 8080,
fetch(req) {
return new Response("Hello Bun!");
},
});
console.log('HTTP server started.');
Run the Bun file:
bun index.ts